Send slack messages from the server for important events
You can send messages on a Slack channel whenever something important happens on the server. For example, new user payment received, some important API threw an error, user submitted some feedback, user was not able to log-in, payment issue, deployment errors, etc so that you could quickly act upon it. Itās also a good way to keep your team notified about it if your team already uses Slack. You can also have different Slack channels to get notified about critical errors, user feedback, etc.
Hereās how it looks:
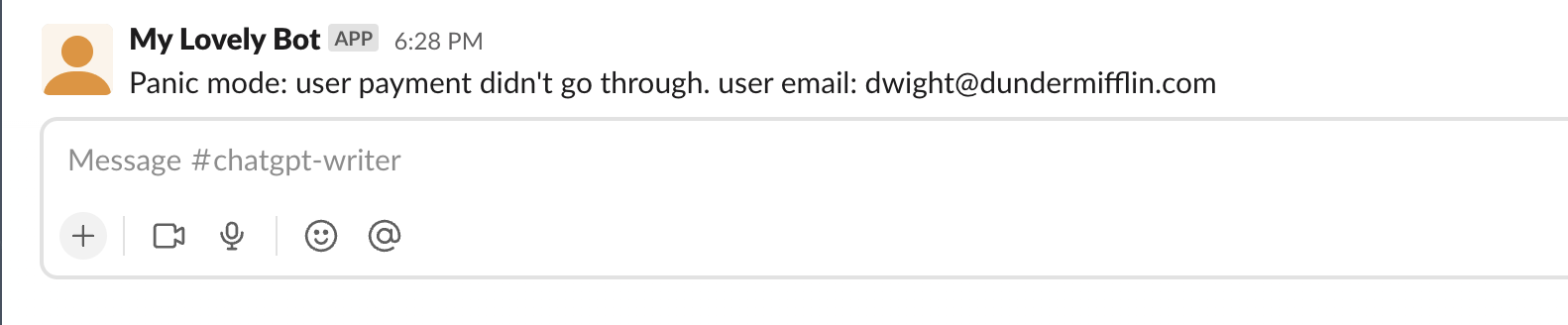
Create a free Slack Bot that would send messages
They call it Slack App.
- Go to https://api.slack.com/apps?new_app=1 and click āCreate New Appā
- Select āFrom Scratchā option ā give App name ā select your workspace
- Go to OAuth & Permissions
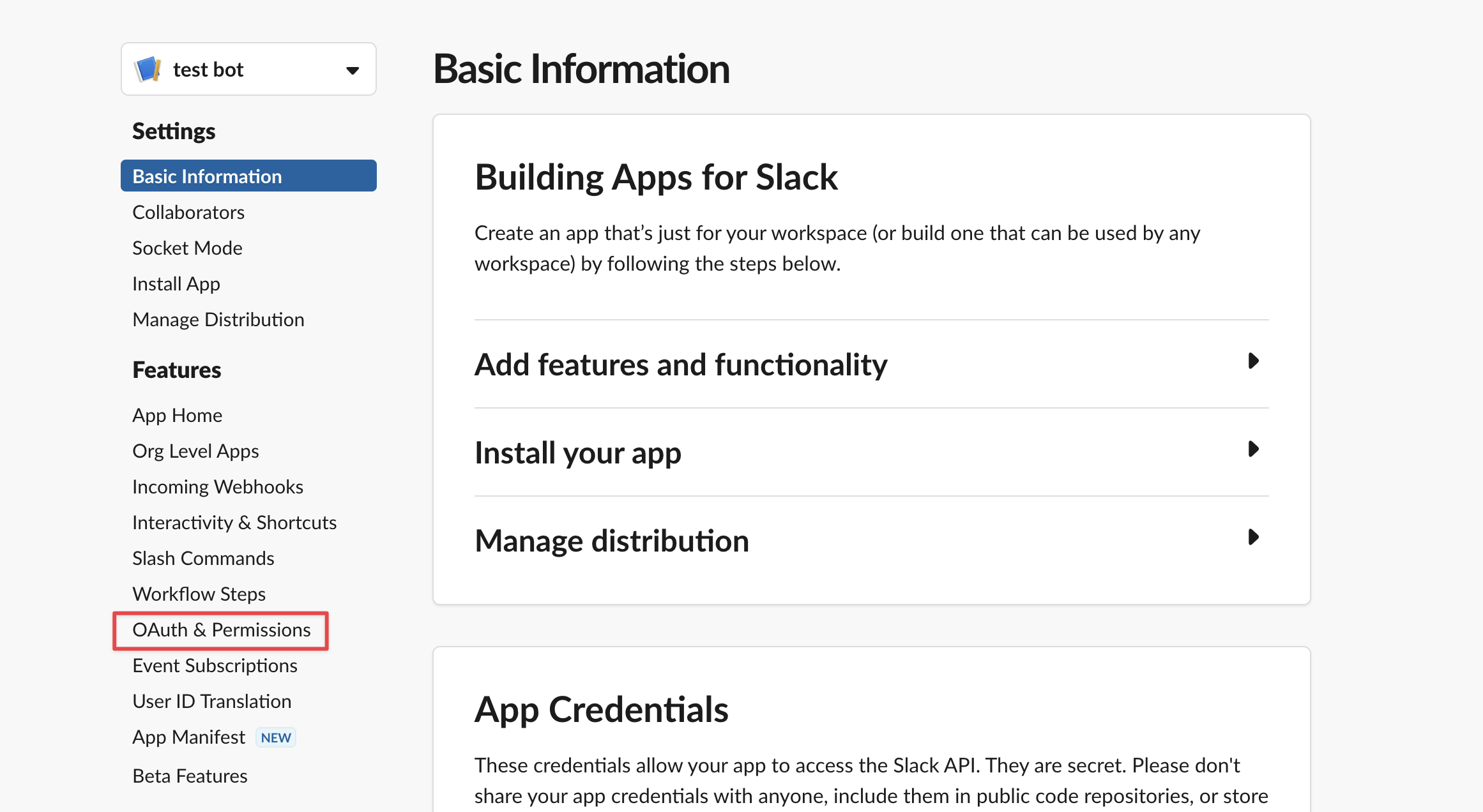
- Go to Scopes ā enter
chat:write
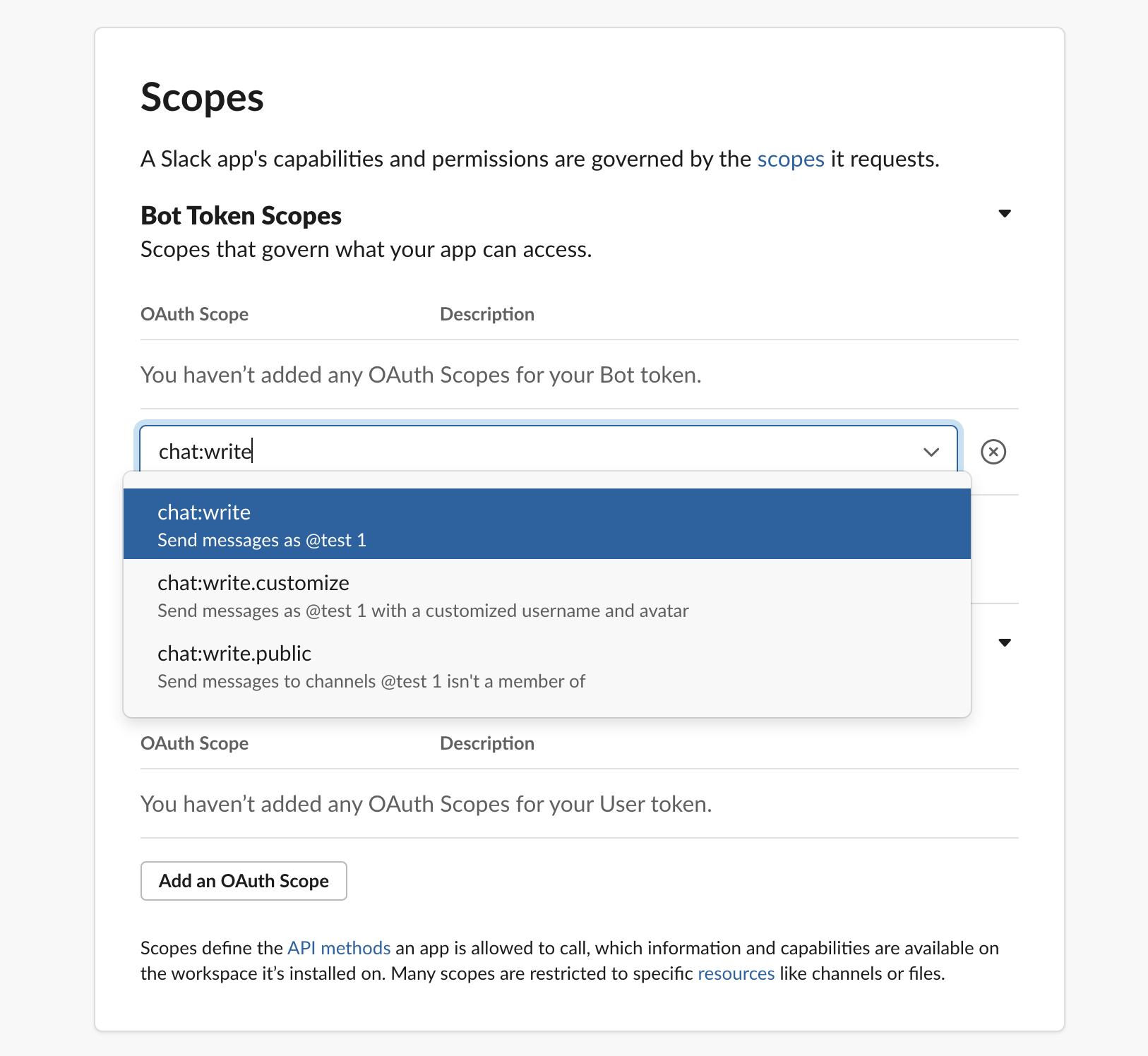
Get Bot Token
Click āInstall to Workspaceā
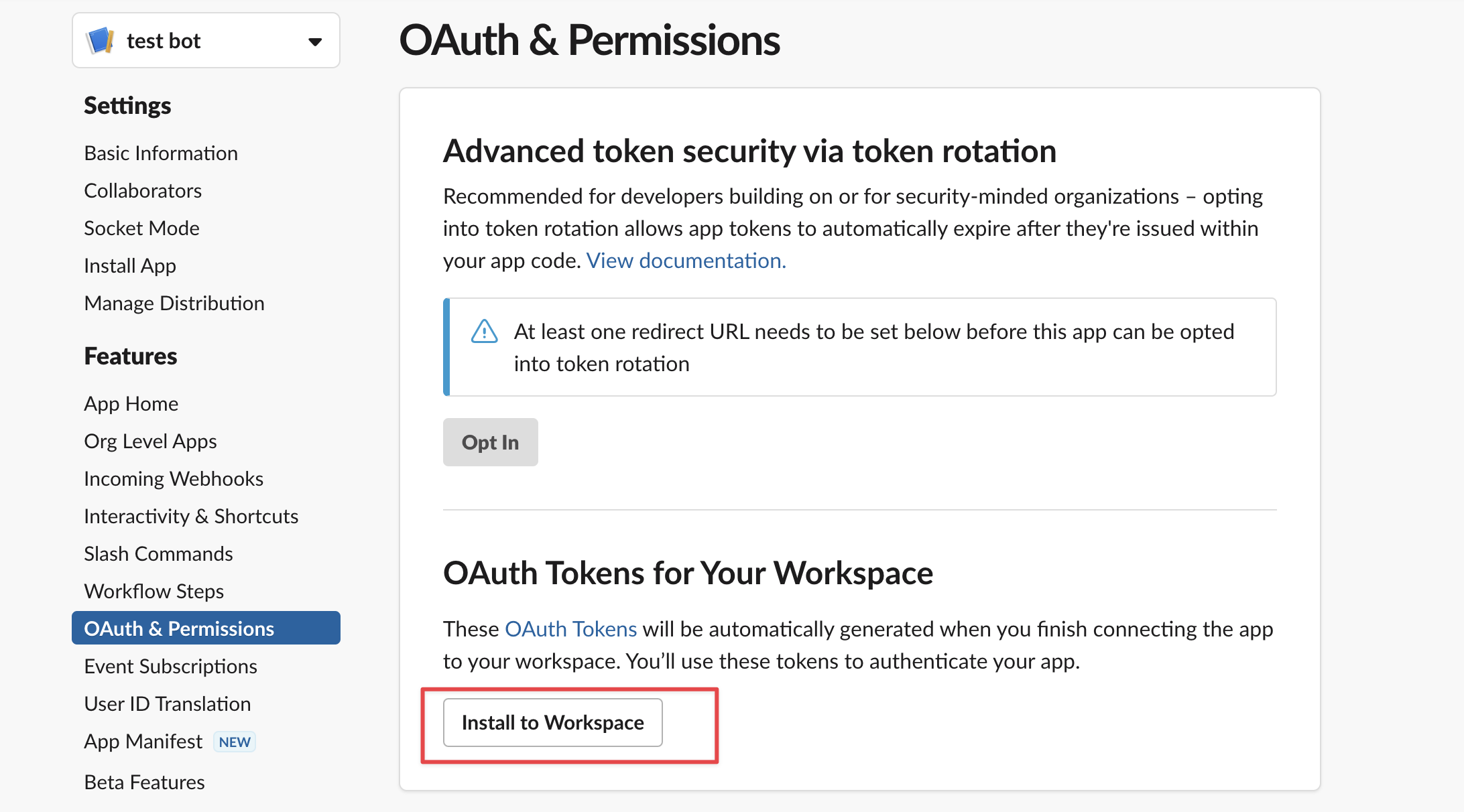
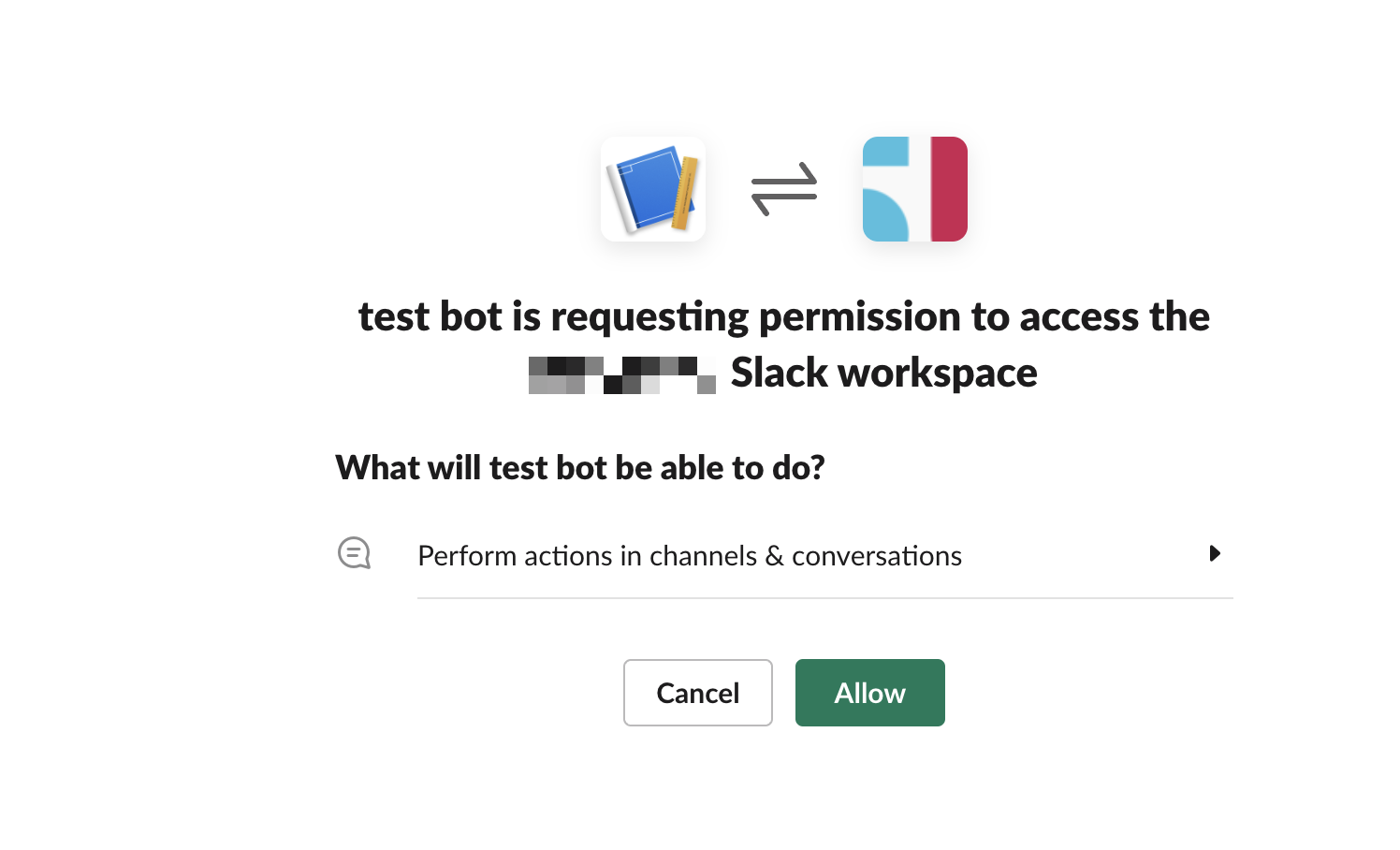
- Copy the Bot token
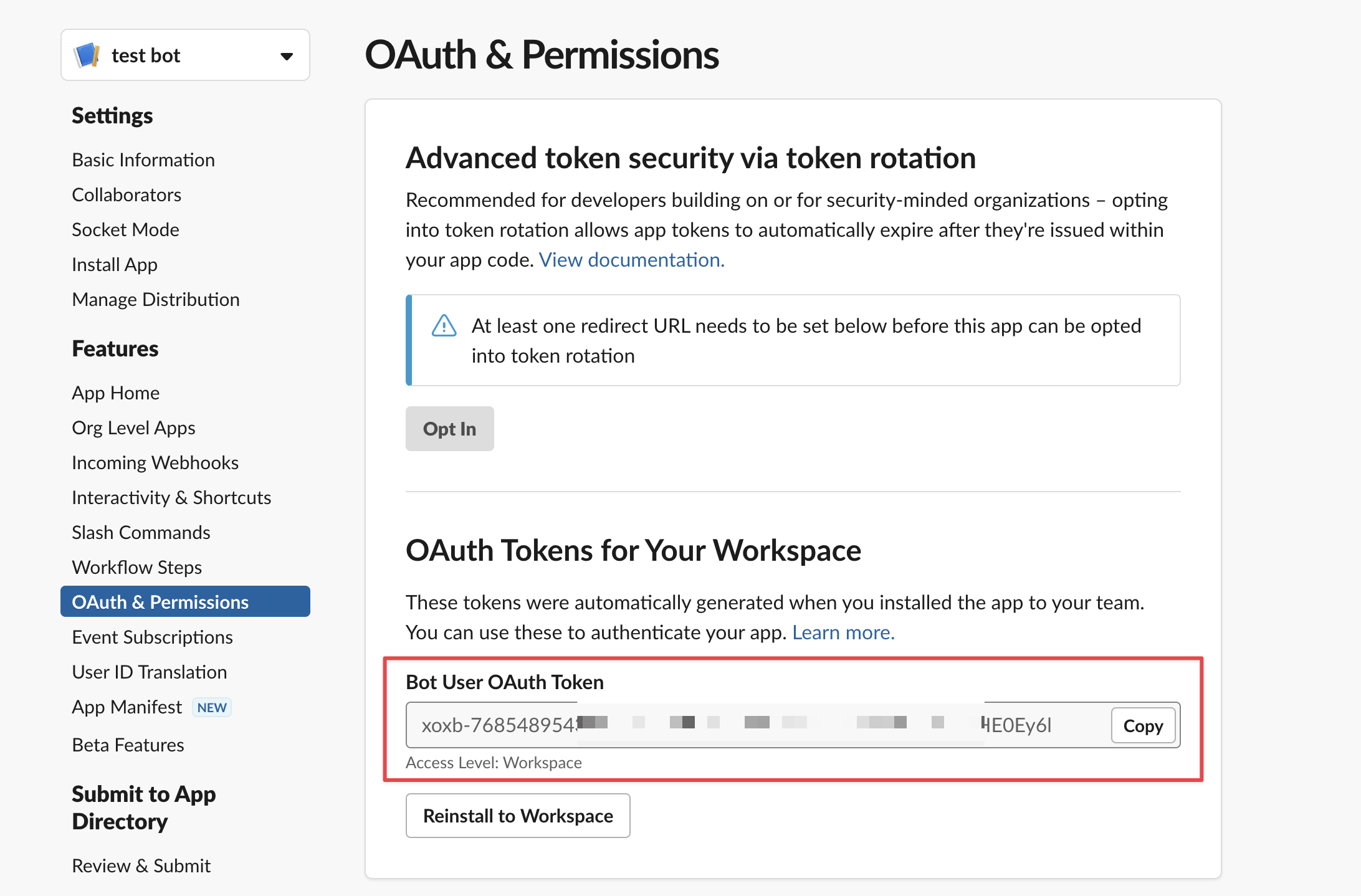
Get Channel ID to post to
Go to Channel info and copy āChannel IDā:
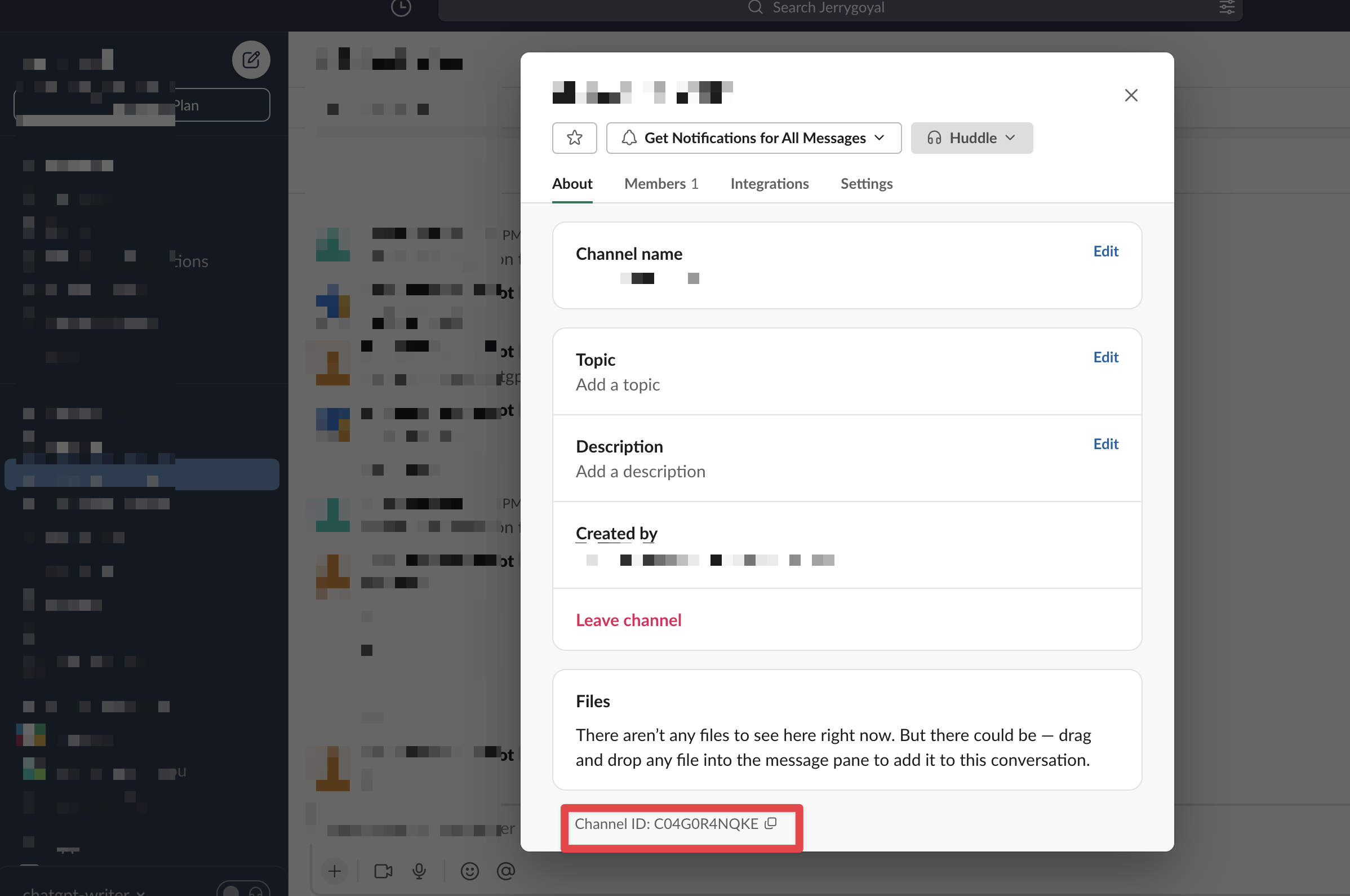
Add Bot to that Channel
Go to Channel āIntegrationā tab and add that Bot as app.
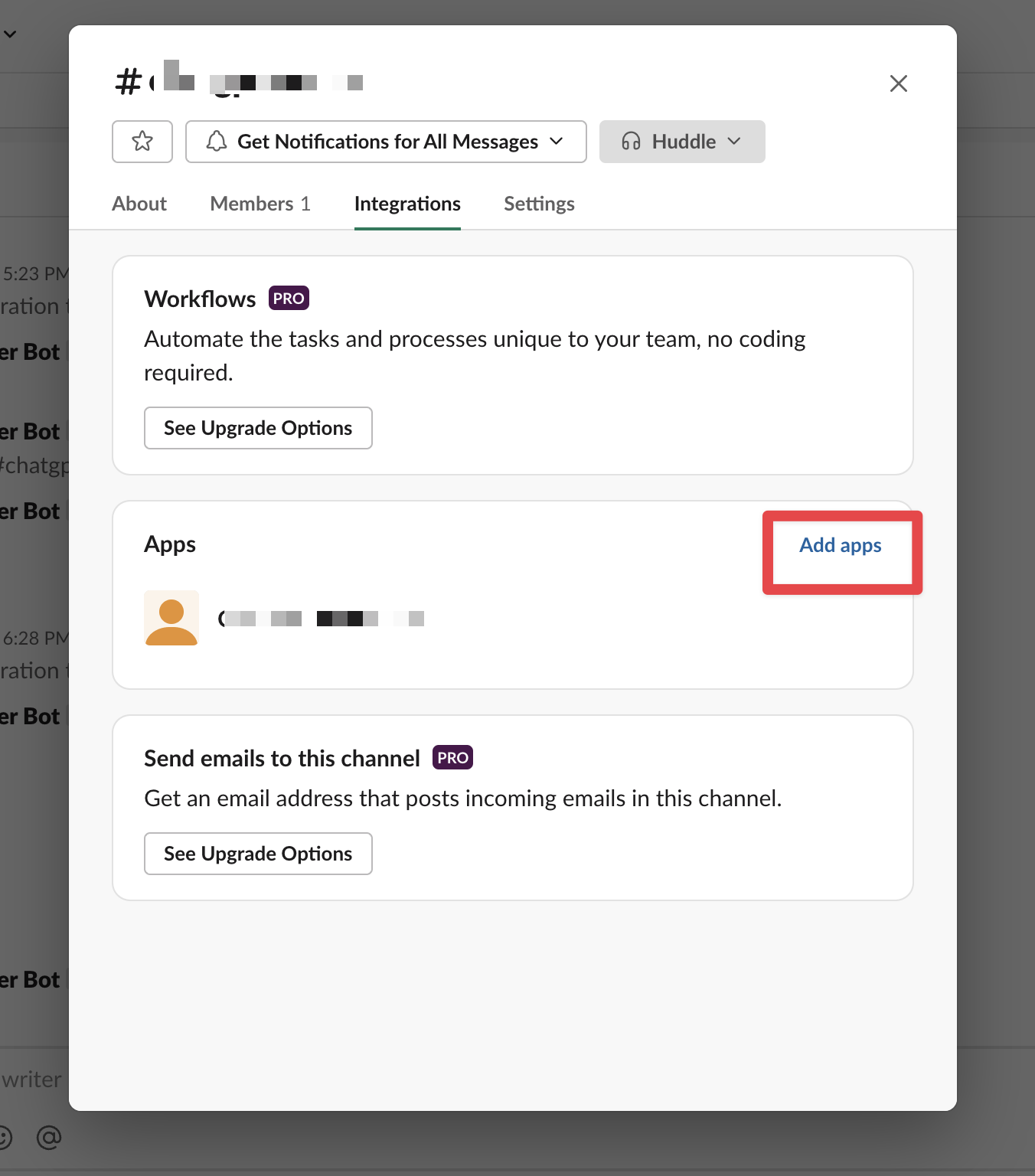
Send message to Slack channel as Bot
Hereās the Typescript snippet for it:
export async function sendSlackMessage(msg: string) {
const response = await fetch("https://slack.com/api/chat.postMessage", {
method: "POST",
headers: {
"Content-Type": "application/json; charset=utf-8",
Authorization: `Bearer ${SLACK_BOT_TOKEN}`,
},
body: JSON.stringify({
channel: CHANNEL_ID,
text: msg,
}),
});
return response;
}